Calculating sales tax is a fundamental requirement in many commercial software applications. In Java, this process involves mathematical operations and leveraging the strengths of Java’s computation abilities. Here, we explore How To Calculate Sales Tax In Java? ensuring accuracy and efficiency in this critical task. This guide is tailored for both beginners and experienced Java programmers seeking to enhance their skills in financial computations using Java.
Key Takeaways
- Understanding the basics of calculating sales tax in Java.
- Implementing sales tax calculation using Java programming.
- Techniques for accurate and efficient tax computation.
- Incorporating Java methods and classes for tax calculation.
How To Calculate Sales Tax In Java?
Calculating sales tax in Java involves several steps where you write a program to compute the tax based on the product’s price and the applicable tax rate. Here’s a detailed explanation of how to do it:
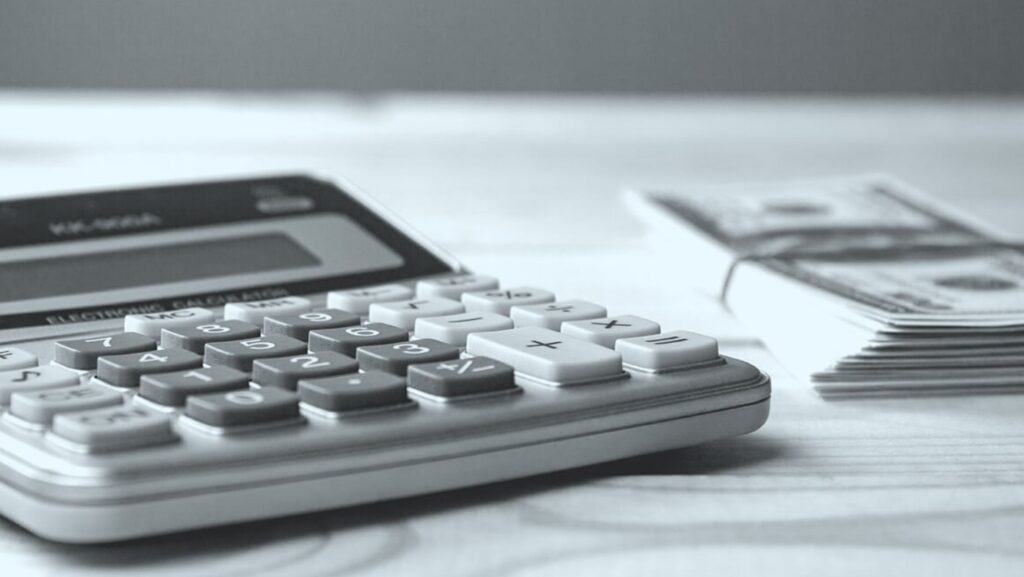
- Understanding the Basics:
- Sales tax is typically calculated as a percentage of the product’s price.
- For instance, if the tax rate is 7% and the product’s price is $100, the sales tax would be 7% of $100, which is $7.
- Setting Up Your Java Environment:
- Ensure you have a Java development environment set up, such as JDK (Java Development Kit) and an IDE (Integrated Development Environment) like Eclipse or IntelliJ IDEA.
- Writing the Java Program:
- Start by creating a new Java class, for example,
SalesTaxCalculator
. - Define variables to store the price of the item and the sales tax rate. You might use
double
for these to accommodate decimal values. Java Code:public class SalesTaxCalculator { public static void main(String[] args) { double price = 100.0; // price of the item double taxRate = 7.0; // tax rate as a percentage } }
- Start by creating a new Java class, for example,
- Calculating the Sales Tax:
- Write a method to calculate the sales tax. This method will take the price and tax rate as parameters and return the calculated tax. Java Code:
public static double calculateSalesTax(double price, double taxRate) { return price * taxRate / 100; }
- In the
main
method, call this function with the price and tax rate and store the result in a variable. Java Code:double salesTax = calculateSalesTax(price, taxRate); System.out.println("Sales Tax: " + salesTax);
- Write a method to calculate the sales tax. This method will take the price and tax rate as parameters and return the calculated tax. Java Code:
- Handling Different Tax Rates:
- If your application requires handling different tax rates for different items, you can pass the specific tax rate for each item when calling the
calculateSalesTax
method.
- If your application requires handling different tax rates for different items, you can pass the specific tax rate for each item when calling the
- Running and Testing the Program:
- Compile and run your Java program.
- Test it with different price and tax rate values to ensure accuracy.
- Enhancements and Real-World Application:
- For real-world applications, consider more complex scenarios like handling different tax rates based on location, integrating with databases to store prices and rates, or creating a user interface for inputting values.
- Error Handling:
- Implement error handling to deal with invalid inputs, such as negative prices or tax rates.
This is a basic overview of calculating sales tax in Java. Depending on your specific needs, you might add more features or handle more complex scenarios.
Sales Tax Calculation Basics
Calculating sales tax in a Java program involves understanding the basics of Java programming and arithmetic operations. Sales tax is typically a percentage of the item’s price. To calculate it, you multiply the item’s cost by the tax rate.
Understanding Variables and Data Types
In Java, variables and data types are crucial for storing the price of an item and the tax rate. For example, double
data type is ideal for representing monetary values due to its precision.
Arithmetic Operations in Java
Java’s arithmetic operators allow for straightforward calculation of sales tax. The multiplication operator (*
) is used to multiply the price by the tax rate.
Implementing Sales Tax Calculation in Java
To implement sales tax calculation, one needs to write a Java method that takes the price and tax rate as inputs and returns the calculated tax.

Writing the Tax Calculation Method
The method calculateSalesTax
can be written, which takes the price and tax rate as parameters. It then returns the product of these two, which is the sales tax.
Example Code for Sales Tax Calculation
Consider an example where the sales tax rate is 7%. For an item priced at $50, the sales tax can be calculated as follows:
Java Code:
public class SalesTaxCalculator { public static double calculateSalesTax(double price, double taxRate) { return price * taxRate / 100; } public static void main(String[] args) { double price = 50.0; double taxRate = 7.0; double salesTax = calculateSalesTax(price, taxRate); System.out.println("Sales Tax: " + salesTax); } }
This code snippet demonstrates a straightforward approach to calculating sales tax.
Advanced Techniques in Sales Tax Calculation
For more complex scenarios, such as different tax rates for different items or locations, Java’s object-oriented features come into play.
Utilizing Java Classes for Different Tax Rates
Creating classes for different types of items or tax rates can make the program more flexible and easier to maintain.
Handling Multiple Items and Tax Rates
For a shopping cart with multiple items, iterating over each item, calculating its tax, and summing up the total tax can be done using Java’s loop structures.
Error Handling and Validation in Tax Calculation
Error handling is crucial to ensure the reliability of the tax calculation process. Input validation ensures that the price and tax rate are within expected ranges.
Implementing Error Handling
Using Java’s exception-handling mechanisms, such as try-catch
blocks, can prevent and manage errors during the calculation.
Validating Inputs
Before performing the calculation, it’s important to validate that the inputs (price and tax rate) are positive and in a realistic range. This can be done using conditional statements.
Sales Tax Calculation in User Interfaces
Integrating sales tax calculations into a user interface, such as a GUI or a web application, enhances user interaction.
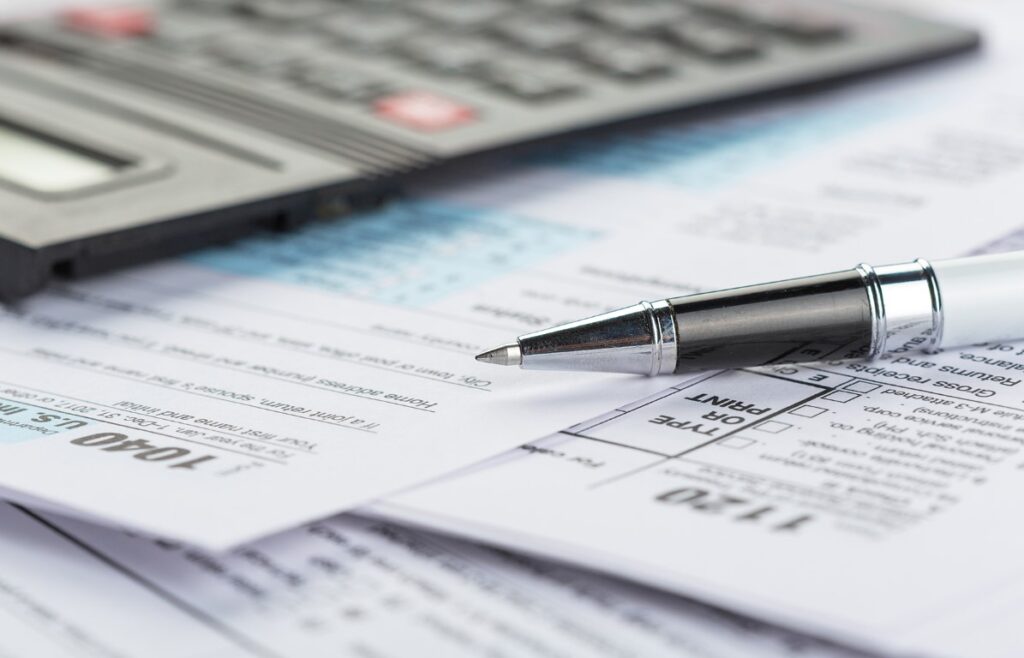
Designing a User-Friendly Interface
Java provides libraries like Swing for GUI development, allowing for the creation of user-friendly interfaces for tax calculation.
Integrating Calculation with UI Components
Linking the backend Java code with frontend elements, such as text fields and buttons, enables real-time sales tax calculation in the user interface.
Optimizing Tax Calculation for Efficiency
When calculating sales tax in Java, optimizing for efficiency is crucial, especially in applications handling a large volume of transactions. Efficient code ensures faster execution and better resource management.
Using Efficient Data Structures
Selecting the right data structures, such as arrays or ArrayLists, can improve the performance of your Java program. These structures allow for efficient storage and retrieval of item prices and tax rates.
Implementing Loops and Algorithms
Efficient looping structures, like for-each loops, can be used for iterating over collections of items. Additionally, implementing well-thought-out algorithms for tax calculation can significantly reduce the computational load and improve performance.
Error Handling and Exception Management
Beyond basic error handling, advanced techniques ensure robustness in Java applications, especially when dealing with user inputs and real-time data processing.
Advanced Exception Handling
Using custom exceptions and handling specific error scenarios, like invalid tax rates or negative prices, can make the program more resilient against data anomalies.
Logging and Debugging
Implementing logging mechanisms helps in tracking the flow of the program and identifying issues quickly. Effective debugging practices are essential for maintaining the accuracy of tax calculations.
Integration with Databases and External Systems
For applications that require storing or retrieving data from databases or external systems, integration is key for seamless sales tax calculation.
Database Connectivity in Java
Java Database Connectivity (JDBC) can be used to connect Java applications with databases, allowing for storing and retrieving prices and tax rates efficiently.
Interfacing with External APIs
In cases where tax rates are dynamic or location-specific, integrating with external APIs to fetch real-time tax rates can be implemented, ensuring up-to-date and accurate tax calculations.
Testing and Validation of Tax Calculation
Thorough testing and validation are imperative to ensure that the sales tax calculation works correctly under various scenarios and inputs.

Unit Testing in Java
Writing unit tests for different components of the tax calculation process helps in identifying bugs early in the development process. Frameworks like JUnit can be used for this purpose.
Validation Techniques
Employing advanced validation techniques, such as boundary value analysis and equivalence partitioning, ensures that the tax calculation logic is robust and handles edge cases effectively.
User Experience and Accessibility
Ensuring that the application is user-friendly and accessible is vital, especially when the end-users are not technically adept.
Designing Intuitive UIs
Creating intuitive and responsive user interfaces that display tax calculations and provide easy navigation can enhance user experience significantly.
Accessibility Considerations
Making sure that the application is accessible, including to those with disabilities, by following best practices in UI design, ensures a wider reach and usability.
Conclusion
Calculating sales tax in Java is a vital skill for developing robust financial applications. Through understanding the basics, implementing methods, and considering advanced techniques, one can effectively integrate sales tax calculations into Java programs. With error handling, input validation, and UI integration, Java developers can build reliable and user-friendly tax calculation features.
People Also Ask
How do you test a sales tax calculation program in Java?
Testing can be done using unit testing frameworks like JUnit, where different scenarios for tax calculation are simulated, and the results are checked against expected values.
Is it possible to calculate sales tax for international transactions in Java?
Yes, Java can calculate sales tax for international transactions by using appropriate tax rates and currency conversion, often through external APIs for real-time data.
How can sales tax calculation in Java be optimized for performance?
Optimize performance by using efficient data structures and algorithms, minimizing the use of heavy loops, and reducing the complexity of the calculations.
What are the best practices for error handling in sales tax calculation?
Best practices include validating input values, using try-catch blocks to handle exceptions, and implementing custom error messages to inform users of any calculation errors.
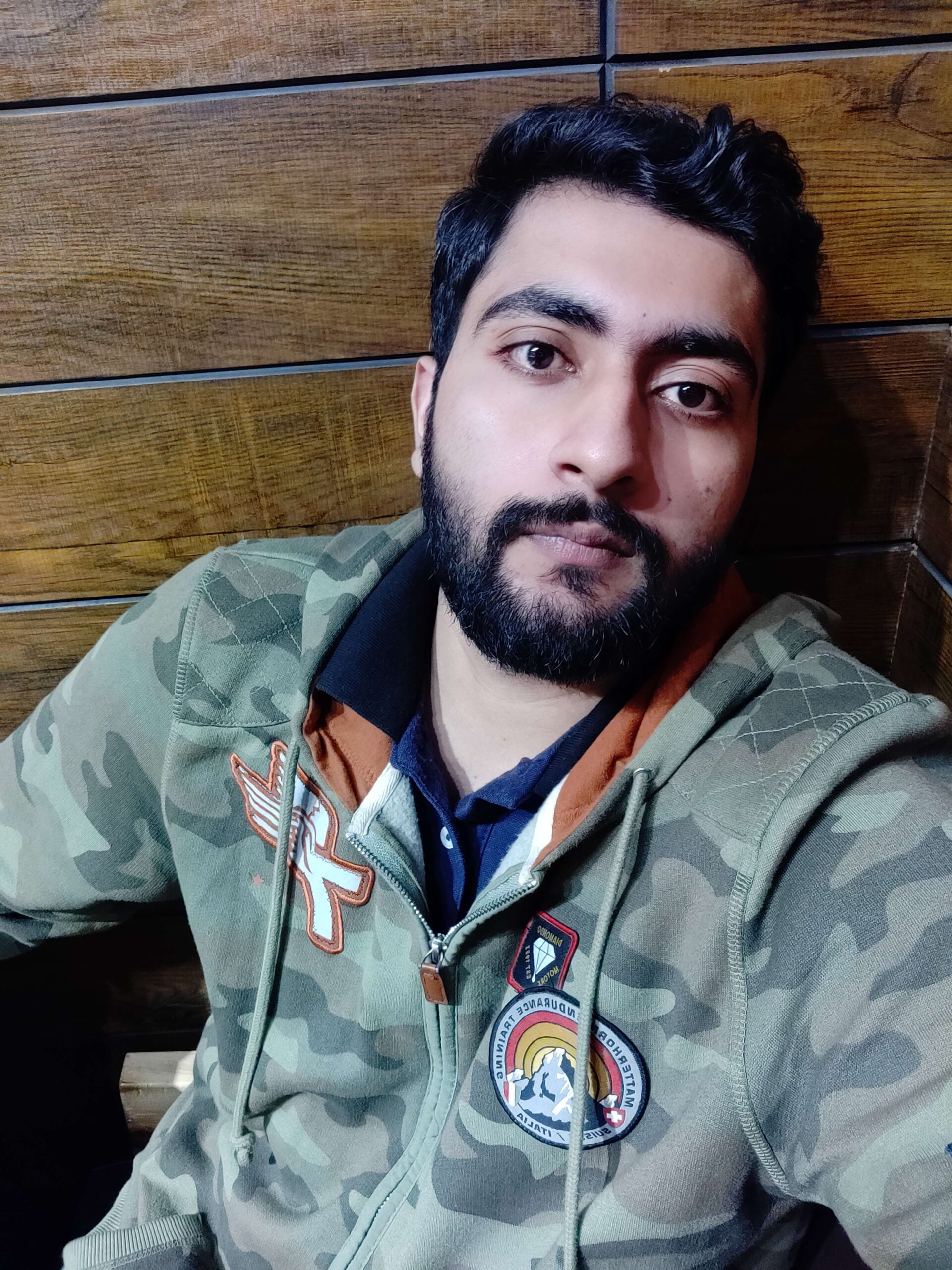
Muhammad Talha Naeem is a seasoned finance professional with a wealth of practical experience in various niches of the financial world. With a career spanning over a decade, Talha has consistently demonstrated his expertise in navigating the complexities of finance, making him a trusted and reliable figure in the industry.